Introduction:
In programming, the memcpy() and memmove() functions are used to copy blocks of memory from one location to another. Although they share similarities, there are important differences in their functionality and usage. This article aims to highlight the distinctions between memcpy() and memmove() to help developers choose the appropriate function for their specific needs.
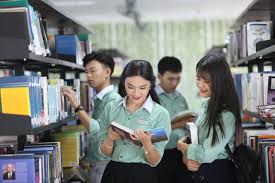
- memcpy():
The memcpy() function is used to copy a block of memory from one location to another. Here are key characteristics of memcpy():
- Memory Copy: memcpy() copies a block of memory from the source address to the destination address. It takes the source address as the first argument, the destination address as the second argument, and the number of bytes to copy as the third argument.
- Overlapping Memory: If the source and destination memory regions overlap, using memcpy() can lead to unpredictable results. Overlapping memory areas can cause data corruption or unexpected behavior. Therefore, it is crucial to ensure that the source and destination memory regions do not overlap when using memcpy().
- Efficient for Non-Overlapping Memory: When the source and destination memory regions do not overlap, memcpy() provides a more efficient memory copy operation. It often utilizes optimized memory copying techniques, such as block copying, to achieve faster results.
- memmove():
The memmove() function is used to copy a block of memory from one location to another, even when the memory regions overlap. Here are important aspects of memmove():
- Overlapping Memory Handling: Unlike memcpy(), memmove() is designed to handle overlapping memory regions. It ensures that the copy operation is performed correctly, regardless of whether the source and destination memory regions overlap.
- Slower Performance: Due to the additional checks and precautions taken to handle overlapping memory, memmove() may be slightly slower than memcpy(). The extra processing overhead ensures the integrity of the copied data when overlapping occurs.
- Safe for All Memory Scenarios: memmove() is a safe choice for all memory scenarios, including situations where the source and destination memory regions may overlap. It guarantees the correct copying of data without risking data corruption or unexpected behavior.
Choosing the Right Function:
- If you are certain that the source and destination memory regions do not overlap, and performance is a concern, memcpy() is a suitable choice. It provides efficient memory copying for non-overlapping memory areas.
- If there is a possibility of overlapping memory regions, or if you want to ensure the correctness of the copy operation regardless of memory overlap, memmove() is the appropriate function. It handles overlapping memory correctly but may have a slight performance impact.
The memcpy() and memmove() functions have distinct purposes and behaviors when it comes to copying blocks of memory. memcpy() is efficient for non-overlapping memory regions and may provide faster performance. However, it does not handle overlapping memory, which can result in unpredictable behavior. On the other hand, memmove() is designed to handle overlapping memory regions and ensures the integrity of the copied data. Although it may be slightly slower, memmove() guarantees the correct copying of data regardless of memory overlap. By understanding the differences between memcpy() and memmove(), developers can choose the appropriate function based on their specific memory copying requirements.