In programming, a memory leak refers to a situation where allocated memory is not properly deallocated or released when it is no longer needed. Over time, this can lead to a gradual depletion of available memory, causing performance issues and potentially crashing the program. In this article, we will explore what memory leaks are and discuss strategies to prevent them.
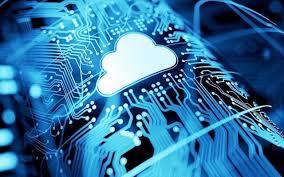
What is a Memory Leak?
A memory leak occurs when dynamically allocated memory is not released, resulting in the loss of memory resources. Here are key aspects of memory leaks:
- Unreleased Memory: When memory is allocated dynamically using functions like malloc() or new, it needs to be explicitly released using free() or delete when it is no longer required. If the release is not performed or is done incorrectly, a memory leak can occur.
- Accumulation over Time: Memory leaks typically occur iteratively, with small amounts of memory being leaked during each occurrence. Over time, the cumulative effect can lead to significant memory loss and degradation of program performance.
- Impact on System: Memory leaks can exhaust available memory resources, leading to system slowdowns, crashes, or even system-wide instability. In long-running programs or those with repeated memory allocations, memory leaks can become critical issues.
Preventing Memory Leaks:
To avoid memory leaks, it is important to follow proper memory management practices. Here are some strategies to consider:
- Allocate and Release Memory Properly: When dynamically allocating memory, always ensure that each allocation is matched with a corresponding deallocation. For example, every call to malloc() should have a corresponding call to free(). Similarly, for C++, every call to new should have a corresponding call to delete.
- Be Mindful of Scope: Allocate memory within the appropriate scope to ensure it is released when it is no longer needed. Avoid allocating memory in a loop or a long-lived scope if it can be allocated outside of it.
- Keep Track of Allocations: Maintain a clear understanding of where and when memory is allocated and deallocated. Use comments or documentation to mark memory allocation and deallocation points, making it easier to identify potential leaks.
- Use Smart Pointers or Garbage Collection: In languages that support it, employ smart pointers or garbage collection mechanisms to automate memory management. These features automatically handle memory deallocation, reducing the chances of memory leaks.
- Test and Debug: Regularly test and debug your code to identify any memory leaks. Use tools and techniques like memory profilers or debugging tools to detect and fix memory-related issues.
- Adopt Best Practices: Follow best practices for coding, such as avoiding unnecessary memory allocations, using appropriate data structures, and minimizing the scope of variables.
Memory leaks can have severe consequences on program performance and system stability. By understanding what memory leaks are and adopting proper memory management practices, developers can minimize the occurrence of memory leaks and maintain efficient memory usage. Allocating and releasing memory correctly, being mindful of scope, tracking allocations, and utilizing language-specific features can help prevent memory leaks. Regular testing and debugging are crucial for detecting and resolving memory leaks in a timely manner. By prioritizing memory management, developers can ensure the stability and efficiency of their programs.